Form Handling
HTML forms and their elements are handled differently by React than other DOM elements. This is because form elements have a natural internal state. For this reason, there are two techniques for handling forms in React. They are known as "controlled components" and "uncontrolled components".
Handling a form submission
Assume we add a simple form to our React application.
return (
<form>
<input type="text" placeholder="Message" />
</form>
)
To respond to the form submission, we can add an onSubmit
attribute and point it to a handler function. The function will receive the event
object as an argument and use the preventDefault()
method to stop the form from causing a page refresh.
function formHandler (e) {
e.preventDefault()
alert('Form submitted.')
}
return (
<form onSubmit={formHandler}>
<input type="text" placeholder="Message" />
</form>
)
Working with uncontrolled components
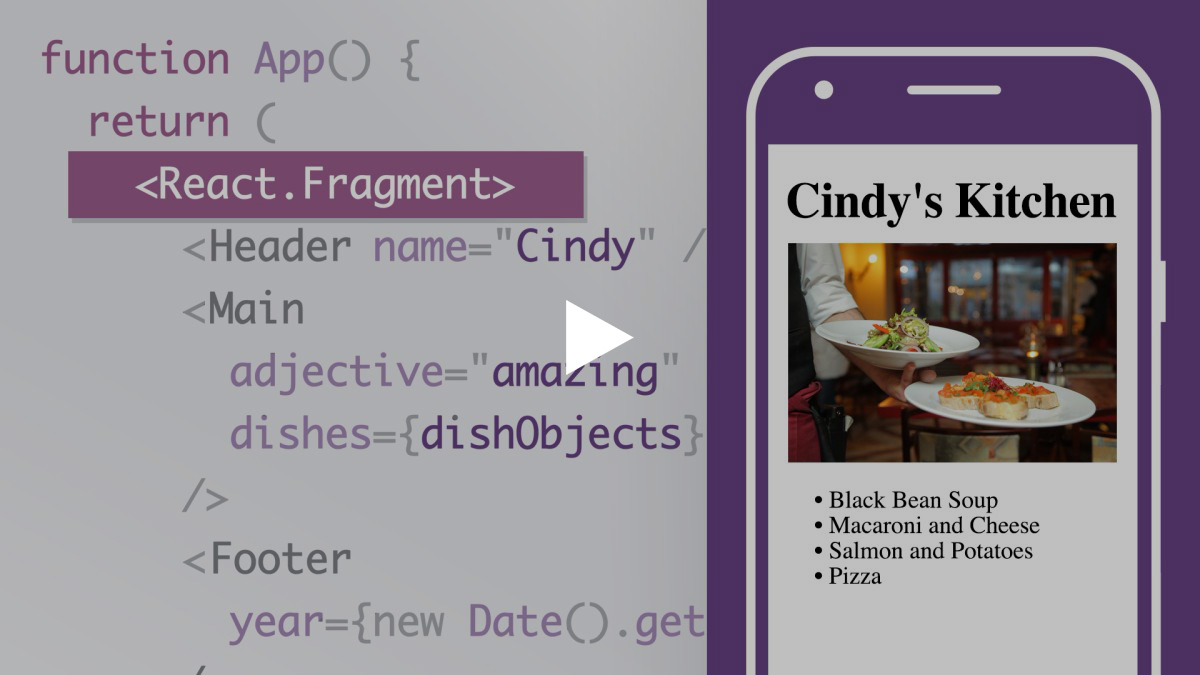
When working with uncontrolled components, form data is handled by the DOM itself instead of React. This is accomplished using the useRef
method. The useRef
method is used to retrieve an individual element and check its value.
To retrieve the value of the input, a reference must be established using the useRef
method. We start by creating a reference variable.
const messageInput = React.useRef()
Next, a ref
attribute is added to the input. The ref
attribute will point to the reference variable.
<input ref={messageInput} type="text" placeholder="Message" />
Now, at any time we can retrieve the input's current value by using the reference variable. The input can be cleared by setting the input's current value.
const messageInput = React.useRef()
function formHandler (e) {
e.preventDefault()
// alert the input's current value
alert(messageInput.current.value)
// clear the input's current value
messageInput.current.value = ''
}
return (
<form onSubmit={formHandler}>
<input ref={messageInput} type="text" placeholder="Message" />
</form>
)
Working with controlled components
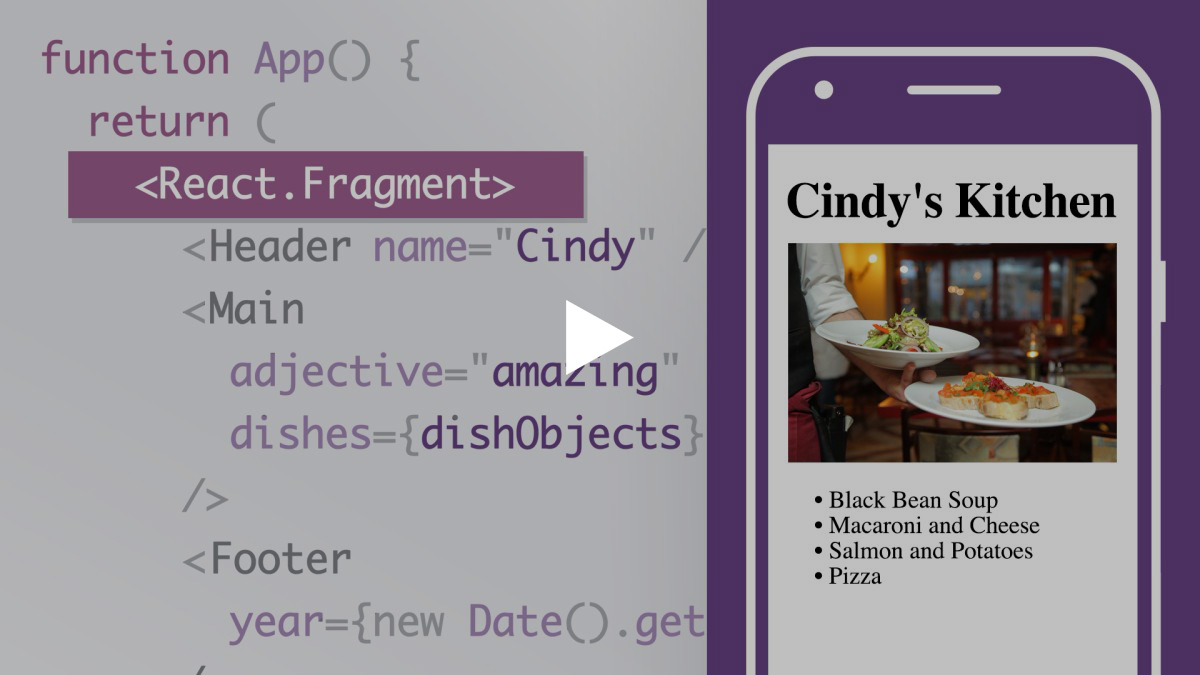
The standard way to retrieve data from a form is using "controlled components". Controlled Components combined the form elements' natural state with the React state by making the React state the "single source of truth".
To use controlled components, we start by declaring a state variable to work with an input.
const [message, setMessage] = React.useState('')
Next, we add a value attribute to the input and point it to the first value of the state variable.
<input value={message} type="text" placeholder="Message" />
Next, we add an event handler attribute to respond when the input changes. The handler function will use the second value of the state variable to update the state variable.
const [message, setMessage] = React.useState('')
function inputHandler (e) {
setMessage(e.target.value)
}
return (
<form onSubmit={formHandler}>
<input value={message} onChange={inputHandler} type="text" placeholder="Message" />
</form>
)
Finally, we can put it all together with the form handler.
const [message, setMessage] = React.useState('')
function inputHandler (e) {
setMessage(e.target.value)
}
function formHandler (e) {
e.preventDefault()
// alert the input's current value
alert(message)
// clear the input's current value
setMessage('')
}
return (
<form onSubmit={formHandler}>
<input ref={messageInput} type="text" placeholder="Message" />
</form>
)