Getting Started
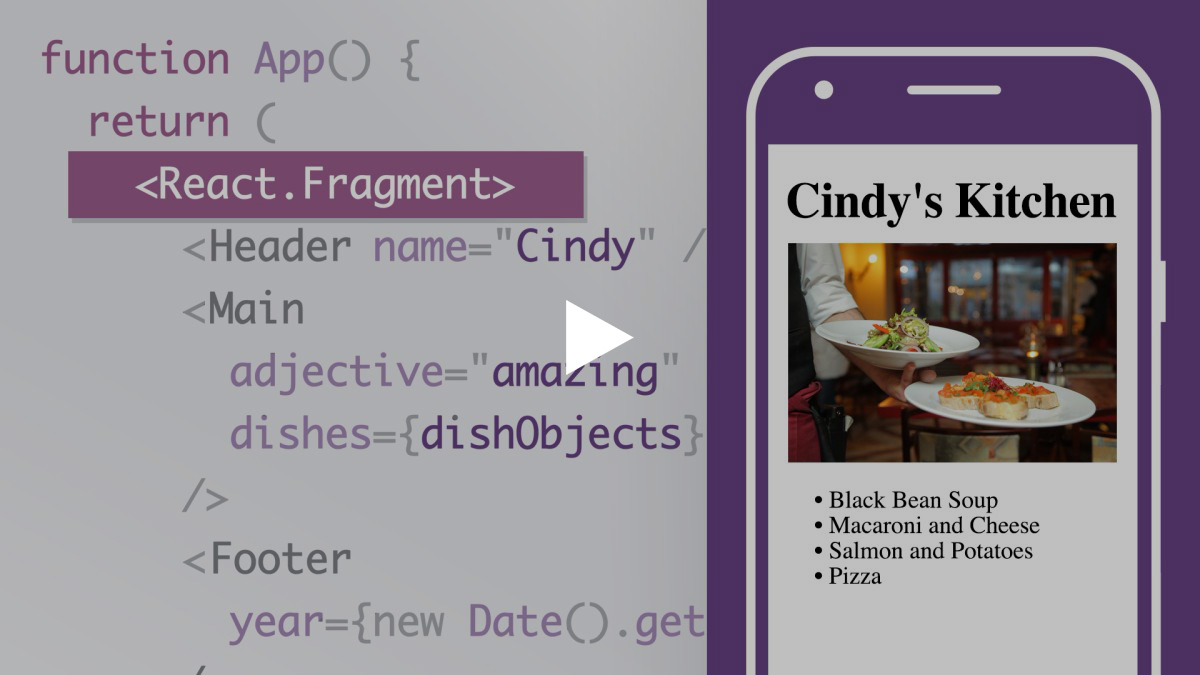
NOTE
The above video was created using React 17. New development patterns have been introduced in React 18.
Adding React
While there are multiple ways to add React to a web page, the easiest way is to use a CDN package.
React.js requires two different script files: react and react-dom. We will insert both scripts using <script>
tags inside the <head>
tag of our HTML.
NOTE
The development version of React.js was used below. The production version should be used when deploying a project.
<head>
<meta charset="UTF-8">
<title>React</title>
<script src="https://unpkg.com/react@18/umd/react.development.js" crossorigin></script>
<script src="https://unpkg.com/react-dom@18/umd/react-dom.development.js" crossorigin></script>
</head>
Creating the React root
Once the scripts have been added, it is possible to start using React. The next step is to add a <div>
to serve as the root of the web application.
<body>
<div id="root"></div>
</body>
Next, we can create the React root, using the ReactDOM.createRoot()
method. The method takes the root element as an argument.
NOTE
The createRoot
method was introduced in React 18 and has replaced the using the render
method directly from ReactDOM
.
<body>
<div id="root"></div>
<script>
const root = ReactDOM.createRoot(document.getElementById('root'))
</script>
</body>
Creating React elements
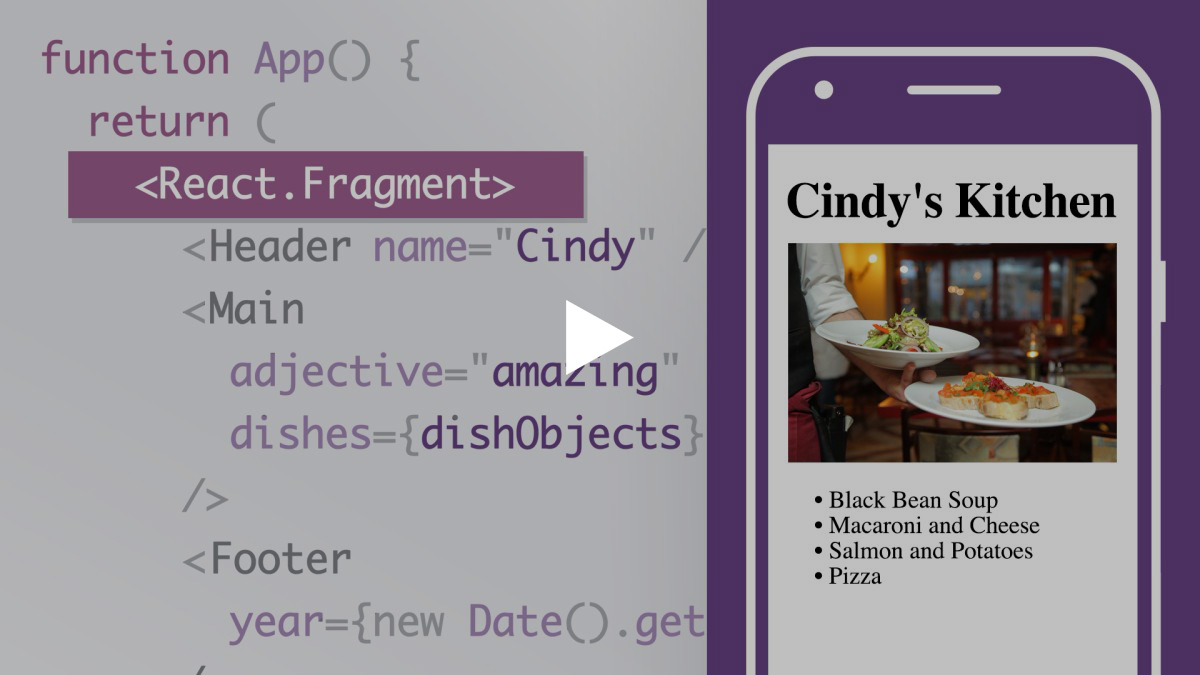
With the React root established, the render
method can now be used to display a react element. We start by creating a new React element using the React.createElement
method storing the results to a variable. Finally, we can pass the variable to the render
method.
<body>
<div id="root"></div>
<script>
const root = ReactDOM.createRoot(document.getElementById('root'))
const heading = React.createElement('h1', null, 'Hello, World')
root.render(heading)
</script>
</body>