Listing Rendering
Working on a web application means working with complex data often store in arrays and objects. This data is then transformed into a list of elements.
Basic list component
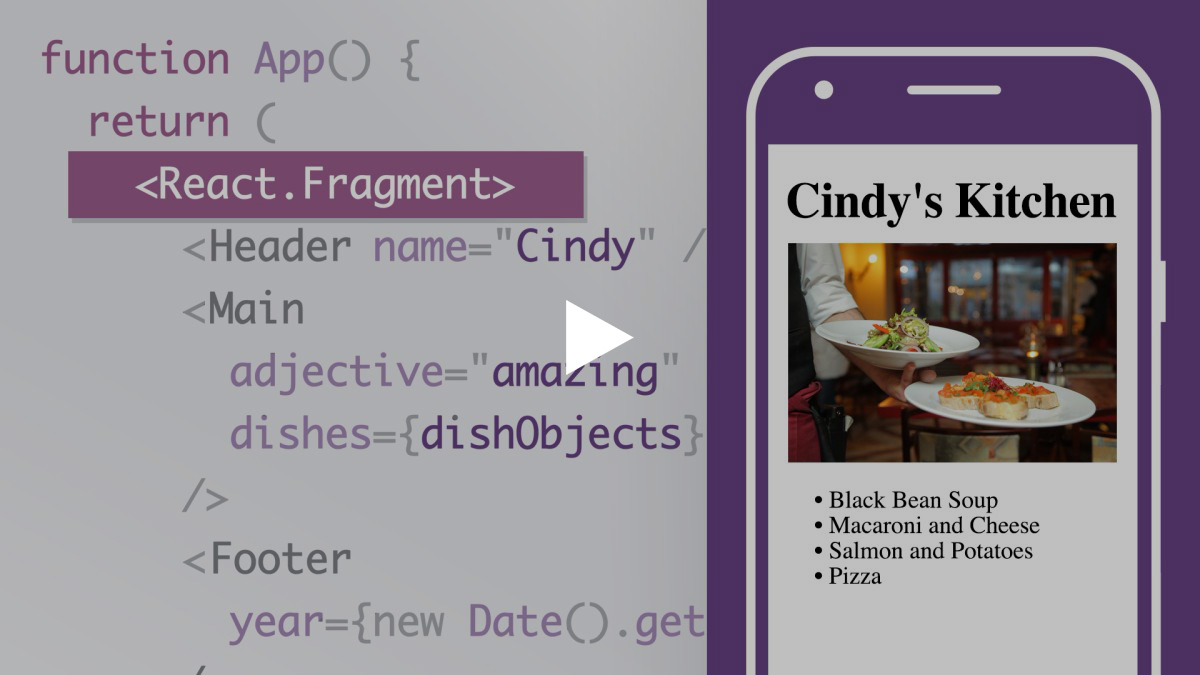
Lists are a key part of working with React. Assume we have an array of colors, which we want to display in an unordered list.
const colors = ['red', 'green', 'blue', 'purple', 'orange']
With the array, we can create a List component that will display a list item for each color in our array.
function ColorList (props) {
const colors = props.colors
const listItems = colors.map((color) => <li>{color}</li>)
return (<ul>{listItems}</ul>)
}
const colors = ['red', 'green', 'blue', 'purple', 'orange']
const root = ReactDOM.createRoot(document.getElementById('root'))
root.render(<ColorList colors={colors} />)
While the code above will create an unordered list of colors, it will also produce a warning that a key should be provided for list items. A "key" is a special attribute that is required when creating a list of elements.
Keys
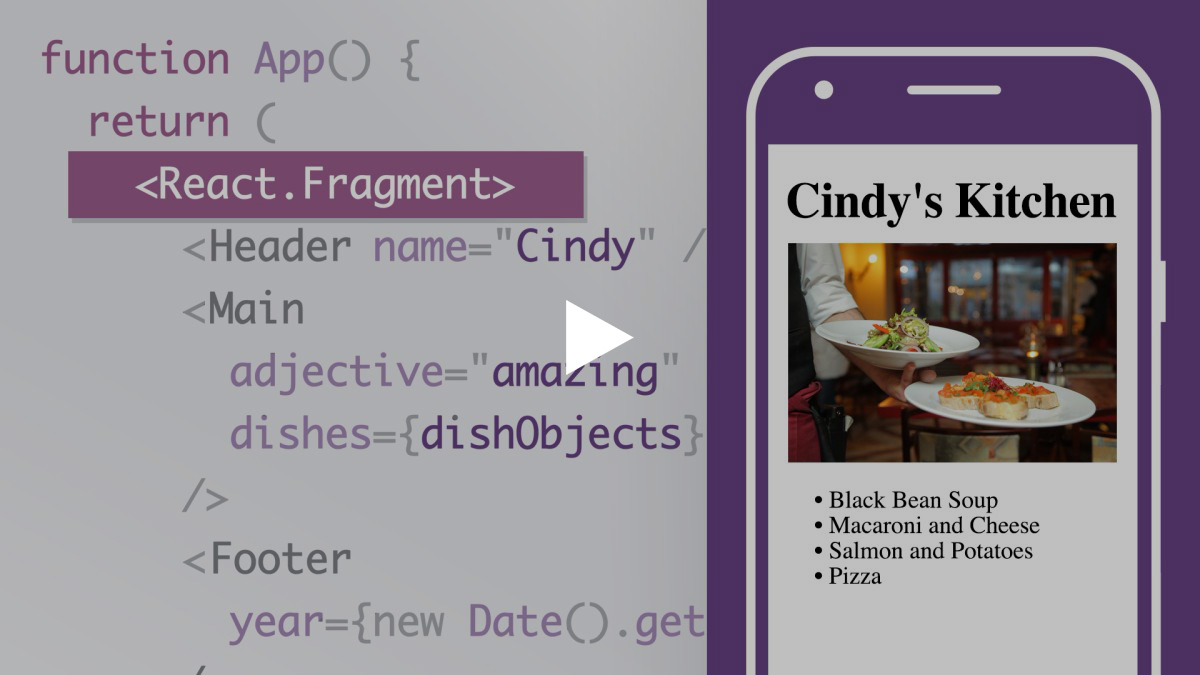
Keys help React identify which items have changed and therefore are required any time a list of elements is created. Keys must be unique and should provide a stable identity for an element.
It is best to choose a unique identifier from the data source. For the example above, we could use the color as the key.
function ColorList (props) {
const colors = props.colors
const listItems = colors.map((color) => <li key={color}>{color}</li>)
return (<ul>{listItems}</ul>)
}
const colors = ['red', 'green', 'blue', 'purple', 'orange']
const root = ReactDOM.createRoot(document.getElementById('root'))
root.render(<ColorList colors={colors} />)
However, this only works if we do not have duplicate color names. For example, the following does produce a warning.
function ColorList (props) {
const colors = props.colors
const listItems = colors.map((color) => <li key={color}>{color}</li>)
return (<ul>{listItems}</ul>)
}
const colors = ['red', 'green', 'blue', 'purple', 'red'] // 'red' listed twice
const root = ReactDOM.createRoot(document.getElementById('root'))
root.render(<ColorList colors={colors} />)
An alternative is to convert each color into an object, containing both a color name and an id. Fortunately, this can be accomplished dynamically using the map()
method.
const colors = ['red', 'green', 'blue', 'purple', 'red'] // 'red' listed twice
const colorObjects = colors.map((color, i) => ({
id: i,
color: color
}))
Now, we only need to update the component to support the color object.
function ColorList (props) {
const colors = props.colors
const listItems = colors.map((obj) => <li key={obj.id}>{obj.color}</li>)
return (<ul>{listItems}</ul>)
}
const colors = ['red', 'green', 'blue', 'purple', 'red'] // 'red' listed twice
const colorObjects = colors.map((color, i) => ({
id: i,
color: color
}))
const root = ReactDOM.createRoot(document.getElementById('root'))
root.render(<ColorList colors={colorObjects} />)