React State
Most web applications support user actions. These actions often cause changes to occur in the application. However, for an application to work properly, it must be able to track these changes through the use of state. State is the data or properties that need to be tracked in an application.
Understanding state
Imagine that we wanted to create a dynamic list that allows the user to add new items. We might create an application like this:
function App () {
const items = [
'Item 1',
'Item 2',
'Item 3'
]
const listItems = items.map(item => <li key={item}>{item}</li>)
const list = <ul>{listItems}</ul>
function buttonHandler () {
items.push(`Item ${items.length + 1}`)
}
return (
<React.Fragment>
<button onClick={buttonHandler}>Add Item</button>
{list}
</React.Fragment>
)
}
const root = ReactDOM.createRoot(document.getElementById('root'))
root.render(<App />)
Now, unfortunately, this will not work as expected. While new items will be added to the items
, they will not be displayed on the page. This is because of how React handles state.
By default, React does not re-render a component when local data is changed. Fortunately, developers can change this behavior by incorporating state into a component. With state, React will listen for changes to local variables and automatically re-render the component. State can be incorporated using the useState
Hook.
Using the State Hook
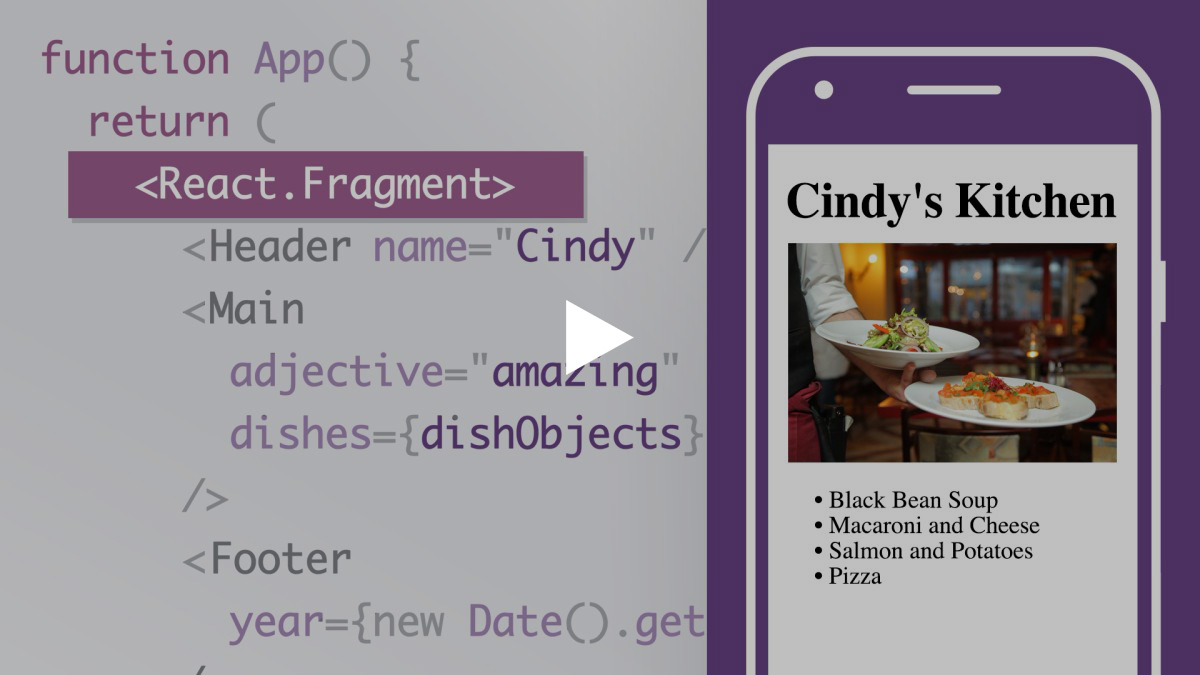
The useState
hook method is used to declare a state variable, which it necessary to create reactive components.
The useState
method returns an array containing two values. The first is an immutable variable holding the value of our state variable. The second is a set function used to update the state variable. When using the useState
Methods to declare the state variable, destructuring is employed to retrieve both the value and the function. The value passed to the useState
method will be the initial value of the state variable.
function App () {
// Declaring a new state variable
const [items, setItems] = React.useState(['Item 1', 'Item 2', 'Item 3'])
Once a state variable has been declared, the value can be retrieved using the first value in the returned array. In the example above, items
is an array, and therefore can be used with the map
method.
const listItems = items.map(item => <li key={item}>{item}</li>)
However, because this value is immutable, the array cannot be altered. Instead, the second value of the returned array. The setItems
function is used to update the items array. This is accomplished by passing the new value as an argument.
setItems([...items, `Item ${items.length + 1}`])
NOTE
Because items
is an array, an array must be passed to the setItems
. When adding a new item to the array, the spread syntax is used.